Selection Sort
Quick Sort Algorithm work on the Principal of Divide and Conquer. It picks an element as pivot and partitions the given array around the picked pivot. There are many different versions of quickSort that pick pivot in different ways.
1. Always pick first element as pivot. 2. Always pick last element as pivot (implemented below) 3. Pick a random element as pivot. 4. Pick median as pivot.
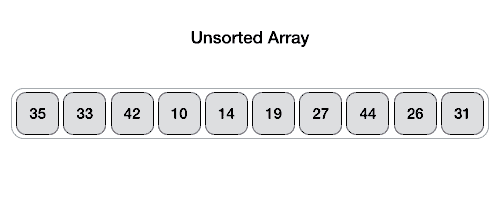
Quick Sort Algorithm Implementation
def pivot_value(data,first,last): pivot=data[first] left = first+1 right = last while True: while left<=right and data[left] <= pivot: left = left+1 while left <= right and data[right] >= pivot: right = right-1 if right < left: break else: data[left],data[right] = data[right],data[left] data[first],data[right] = data[right],data[first] print("for step how to run") print(data) return right def quickshort(data,first,last): if first<=last: a = pivot_value(data, first, last) quickshort(data, first, a - 1) quickshort(data, a + 1, last) data=[10,6,5,4,3,2,1] b=len(data) quickshort(data,0,b-1) print(f"Final Ans : {data}")
Quick Short Algorithm Analysis
Best Case: O(nlogn) Average Case: O(nlogn) Worst Case: O(n^2) Space Complecity: O(logn) Quick Short Algorithm are Internal Shorting Algorithm Quick Short Algorithm are Recursive Shorting Algorithm Stability: No Quick Short Algorithm Are Not Stable Algorithm Adpative: Yes Quick Short Algorithm Are Adpative Algorithm